Overview:
DoctorOnCall is a Java sample application intended for doctors with long-term patients and have to track their patients' appointment details as well as particulars. This application was developed by team T11-2 of CS2113T in National University of Singapore.
Features implemented: Refer Patient (major/ main feature), length (minor feature)
-
Major enhancement: added a refer command that allows user to directly refer a patient within the addressbook and automatically update the relevant particulars
-
What it does: Allows user to do a direct patient refer to another doctor
-
Justification: On the same database where patients' particulars are stored, having this features allows for a quick and easy way to refer patients without the need to swap to another dsatabase/ update the addressbook manually.
-
Highlights: The challenge of this implementation is to analysize existing commands - Add, Delete and Find - understand the concepts behind these working commands and extracting only the relevant parts to make this feature possible.
-
-
Minor enhancement: added a length command that returns the nuber of entries in the addressbook
-
What it does: Allows user to query the number of entries without having to see the full list with every patient’s particulars (when using list command).
-
Justification: Users would like a quick access to how many patients they have. This feature hides all other information and only outputs the number of entries.
-
Highlights: The challenge of this implementation is understanding how the command list works and modelling its implementation and only getting the parameteres required (size).
-
-
Other contributions:
-
Project management: Managed releases v1.1 - v1.4, as well as raise and resolve issues on issues tracker.
-
Documentation: Did cosmetic tweaks to original template by formatting Use Cases for the existing commands of the Developer Guide as well as the DeveloperGuide’s design section. For my contributions to the Design Section, please refer to https://github.com/cs2113-ay1819s2-t11-2/main/blob/master/docs/DeveloperGuide.adoc#design
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Getting length of the address book : length
Shows the number of entries in the address book at the point of query.
Format: length
Referring a patient to a doctor: refer
Refers a patient from the address book to a doctor.
Note: This command is overloaded with two formats.
Format 1: refer NAME [MORE_NAMES]
Refers target patient to a default doctor and shows a success message with patient’s updated particulars if there is only one unique entry. If there are multiple entries, all patient entries with the target keywords in the addressbook will be printed and user will be prompted to identify the correct patient. If target patient does not exist in the addressbook, an error message will be printed.
Default doctor is the last specified doctor or Dr Seuss
if never specified before previously.
Examples:
* refer John
* refer John Doe
* refer Doe
Format 2: refer d/ DOCTORNAME p/NAME [MORE_NAMES]
Refers target patient to a doctor specified by the user. Format 2 works exactly like format 1. If input contains an invalid doctor name (non-alphanumeric), an invalid command format message will be printed.
When a refer is successful using this format, the default doctor would be updated as this new doctor specified in the latest format 2 entry.
Examples:
* refer d/DoctorTan p/John Doe
* refer d/Dr Tan p/John Doe
* refer d/Dr Tan p/Doe
Contributions to the Developer Guide:
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
For my contributions to the Design Section, please refer to https://github.com/cs2113-ay1819s2-t11-2/main/blob/master/docs/DeveloperGuide.adoc#design
Refer feature
Current Implementation
The refer feature is facilitated by the ReferCommand class, Logic class, Parser class and AddressBook class, and it implements the following operations:
-
prepareRefer()
— checks if input is valid and splits it at the specific prefix d/ when necessary -
getPatientToRefer()
— looks up addressbook and returns target patient to refer -
toRefer = new Person( person.getName(), person.getPhone(), person.getEmail(), person.getAddress(), person.getAppointment(), new Doctor(referraldoctor), person.getStatus(), person.getTags() );
— Copies and modifies taget patient’s doctor name -
tagSet.add(new Tag("referred"))
— adds a refer tag -
addressBook.removePerson(person)
— removes target patient -
addressBook.addPerson(toRefer)
— adds the newly update patient entry back into the addressbook
Given below is an example usage scenario and how the refer feature is incorporated at each step. The Sequence Diagram for referring a patient is being provided at the end to further aid in illustrating this example.
Step 1. The user executes command "refer john"
Step 2.Logic class calls Parser class through command parserCommand("refer John")
Step 3. Parser class calls method prepareRefer("John")
to checks if input is valid and splits it at the specific prefix d/ when necessary.
Step 4. Parser class calls ReferCommand to start evoke the refer.
Step 5. ReferComand class calls method getPatientToRefer("John")
to looks through the Addressbook for all entries containing the keyword "john"
Step 6. If there is only one entry, ReferCommand modifies the entry’s Doctor and adds a new tag 'refer' to the current collection of tags, deletes the old and adds the newly modified patient entry into the addressbook. If there are multiple entires, ReferCommand class prints all entries in the address book with the keyword and prompts user to key unique keyword (Repeats to Step 1).
Step7. The successful execution returns a MESSAGE_SUCCESS along with the patient’s updated particulars.
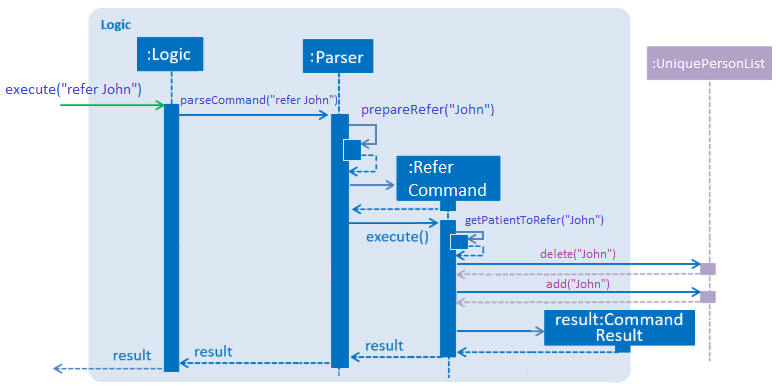
Length feature
Current Implementation
The refer feature is facilitated by the LengthCommand class, Logic class, Parser class and AddressBook class, and it implements the following operation:
-
addressBook.size()
— queries and returns the size of the addressbook
Given below is an example usage scenario and how the length feature is incorporated at each step. The Sequence Diagram for getting addressbook length is being provided at the end to further aid in illustrating this example.
Step 1. The user executes command "length"
Step 2.Logic class calls Parser class through command parserCommand("length")
Step 3. Parser class calls LengthCommand to query the size of addressbook.
Step 4. The successful execution returns the length of the address book.
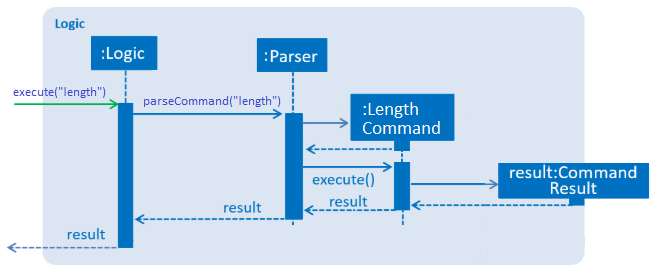
=== Use case: UC03 - Refer patient Main success scenario: . The user requests to refer patient to another doctor. . AddressBook finds patient from the list of entries, and extracts all the relevant particulars of the target patient. . Addressbook updates the extracted particulars with the new doctor’s name and patient tag. . AddressBook deletes the old patient entry from the main list and adds the newly updated one into the main list. + Use case ends. Extensions * 1a. The format entered by the user is incorrect. 1a1. AddressBook shows an error message and prints the format for user to follow. 1a2. User re-enters new patient’s particulars. * Steps 1a1 - 1a2 are repeated until the correct format is entered by the user. + Use case resumes at step 2. * 1b. There are multiple entries in the addressbook with the same patient name(s) entered by the user. 1b1. AddressBook prints out all similar patient entries and prompts users to to identify the correct patient. 1b2. User enters correct patient’s full name. + Use case resumes at step 2. * 1c. The format of the doctor’s name entered by the user is incorrect (non-alphanumeric). 1c1. AddressBook shows an error message and reminds users that doctor names can only contain alphanumeric characters. 1c2. User enters a valid doctor’s name that follows the condition above. + Use case resumes at step 2. === Use case: UC06 - Length of address book Main success scenario: . User requests for length of address book. . AddressBook gets the current length and prints out the number of entries. + Use case ends. Use case ends. === Refer feature [NOTE] Please ensure the following entries have been added into the addressbook before trying the test cases below. If there are not entries in the addressbook, the 'no such person' execption will be shown. . refer NAME [MORE_NAMES] .. Prerequisites: please add the following patient entries being proceeding: .. Test case 1: .. Test case 2: .. Test case 3: .. Test case 4: .. Test case 5: .. Test case 6: .. Test case 7: === Length feature . length .. Prerequisites: NIL .. Test case 1: .. Test case 2: .. Test case 3: |